Angular 6.1 — small changes yet mighty
Angular 6.1 just hit NPM a few days ago and we, the Angular developers loved it. The update included a lot of bug fixes and patches along…
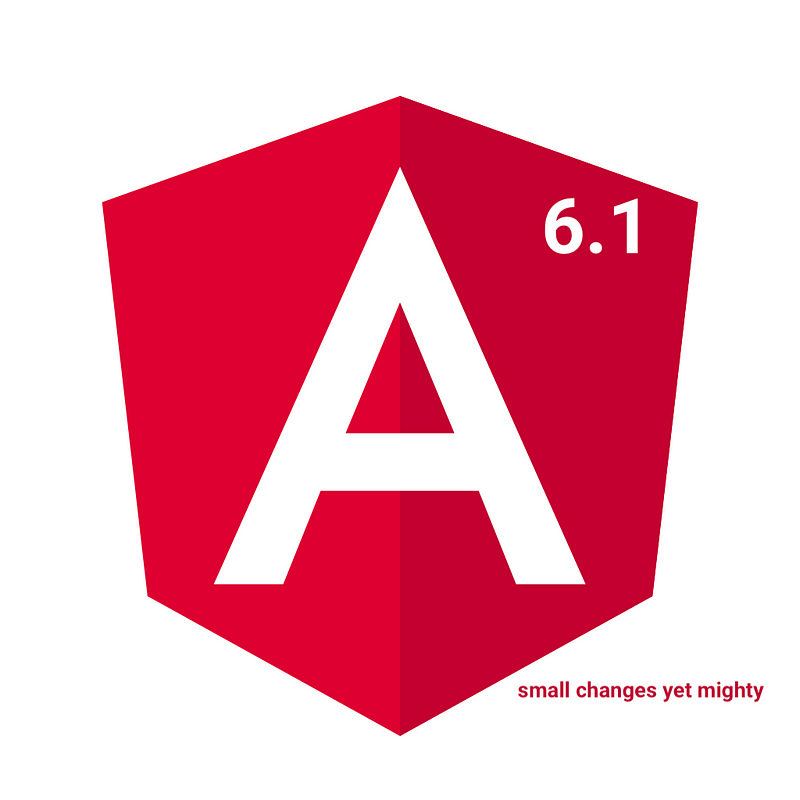
Angular 6.1 just hit NPM a few days ago and we, the Angular developers loved it. The update included a lot of bug fixes and patches along with some really cool features that will make your everyday Angular development easier. You can see the release here.
The release included a lot of minor patches but we will go through some of cool features that really caught my eye. They may not be a huge thing like Angular Elements or Ivy, but again, great things come in small patches (pun intended). Let’s go through the features that would make your lives easier.
Scroll position restoration with routing
Angular has done something in 6.1 that we probably had to do ourselves in most applications that we built earlier with Angular. Whenever we routed to a different page, the scroll position remained the same. See the example (& code) below that shows the behavior:
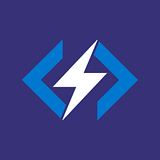
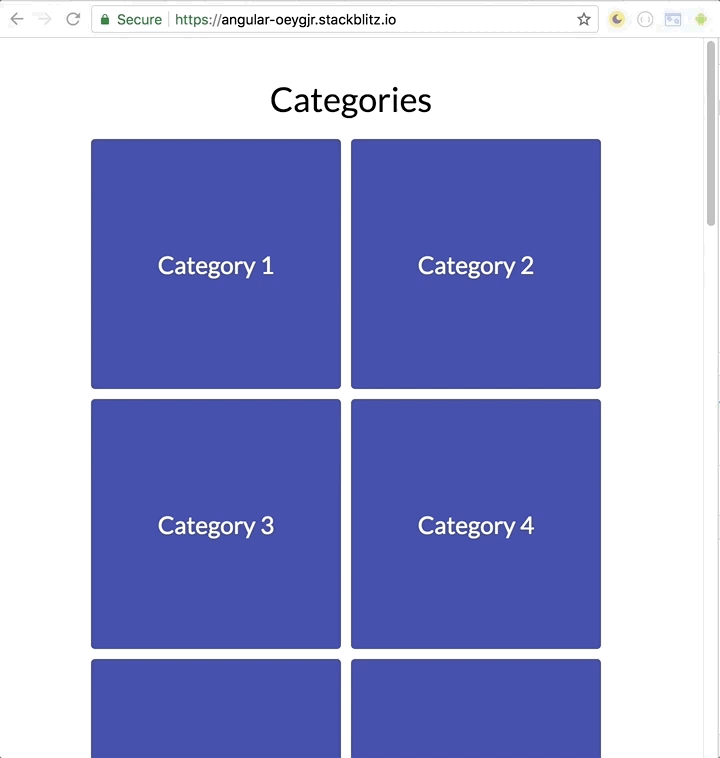
Notice that when we navigate from categories page to the products page, the page doesn’t scroll automatically to the top and retains its position.
`scrollPositionRestoration` to the rescue. You can now pass extraOptions
to your RouterModule.forRoot()
as a second parameter and can specify scrollPositionRestoration: enabled
to tell Angular to scroll to top whenever the route changes.
See the modified version below with the updated packages to 6.1.0:
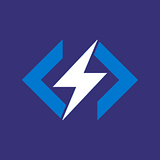
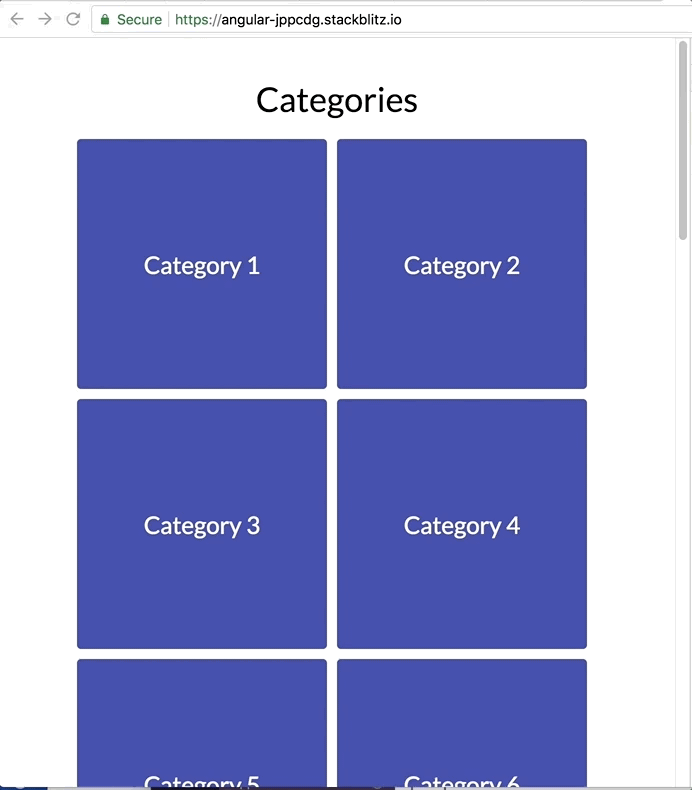
The ‘keyvalue’ pipe
This is one of those things that you wished were built-in into the framework. Perhaps we all have been there when we accidentally or intentionally provided an object to *ngFor
. Angular then got angry and said:

Now you can use your object or a Map using the keyvalue
pipe introduced in Angular 6.1 to display key/values on the view (template).
To use the keyvalue
pipe, simply add it with a pipe on the template to your model as below:@Component({
selector: 'my-component',
template: `
<div *ngFor="let prop of myObject | keyvalue">
<div>key: {{prop.key}}</div>
<div>value: {{prop.value}}<div>
</div>
`
})
export class MyComponent {
myObject = { firstName: 'Ahsan', lastName: 'Ayaz'};
}
I’ve also created a demo to show some examples that show the usage of thekeyvalue
pipe to iterate over objects and Map(s). Link below:
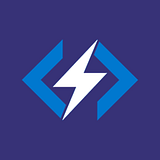
Shadow DOM (v1) Support
Angular provides CSS scope encapsulation which limits the css styles to be applied only to the component. Until 6.1, there were three encapsulation modes:
- Native — Uses shadow DOM v0 and encapsulates style within components
- Emulated — This is the default mode. Angular adds a host element attribute to the template elements and uses the
styles
orstyleUrls
to encapsulate the styles using that host element attribute selector. - None — This means we’re telling Angular to not use any styles encapsulations
You can read more about Angular’s ViewEncapsulation here.
Angular 6.1 introduced a new mode ShadowDom
which uses modern Shadow Dom (v1) to encapsulate styles which is supported by most of the major browsers now. You can simply use it as follows:@Component({
selector: 'my-component',
templateUrl: './my-component.html',
encapsulation: ViewEncapsulation.ShadowDom
})
class MyComponent {}
TypeScript 2.9
Angular supported Typescript 2.7 but since 6.1, Angular supports TypeScript 2.8 as well as 2.9.
To see a complete list of bug fixes & new features, please see the CHANGELOG.
If you know someone who would find this article informative, please share! And follow me on Twitter to stay in touch.